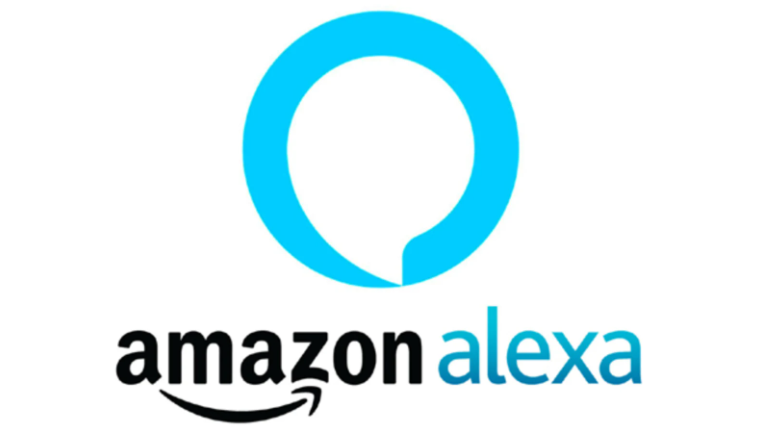
When creating an Alexa skill, one of the best options is to use and Alexa Hosted Skill, which will allow you to have all the necessary resources to develop directly inside the Alexa Developer console and will automatically provision all the basic cloud resources used by Alexa, such as Lambda Endpoints, S3 bucket, DynamoDB table and AWS CodeCommit repository.
The below steps will guide you through the creation of your first skill with the addition to add a small feature which will allow Alexa to connect to a live API and speak back the information to you.
Login to the Alexa Developer Console, if you do not have an account create one - https://developer.amazon.com/alexa/console/ask
In the following initial page, click on Create Skill
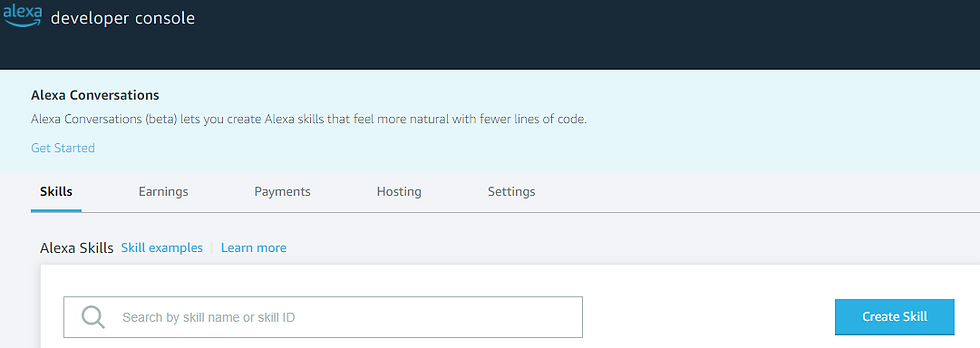
Fill out the desired Alexa skill name
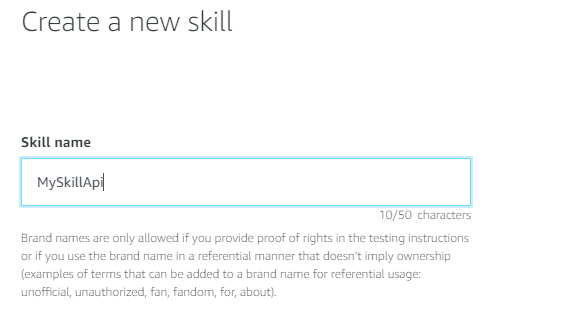
When choosing a model, select the Custom option this will allow you customize the experience and create your own interactions. The customized model will provide ways to define how the user will interact (spoken input) to the intents handled by your cloud service.
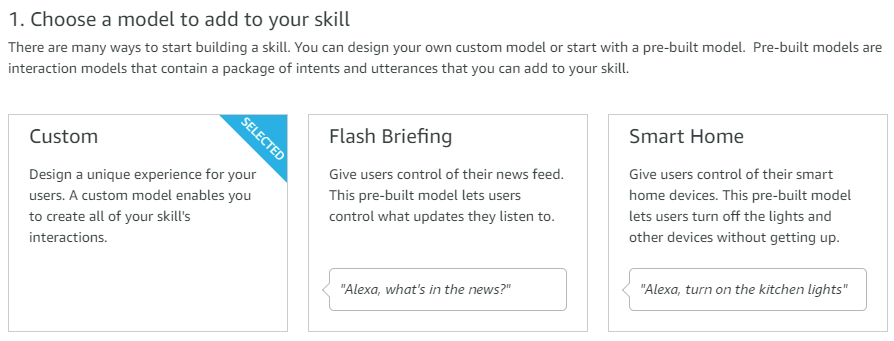
Select the Alexa-host, as explained above this will do all the necessary provision for you the developer. We are choosing Node.js for codification but the same can be achieved using Python. Both languages are supported by Alexa-hosted skills.
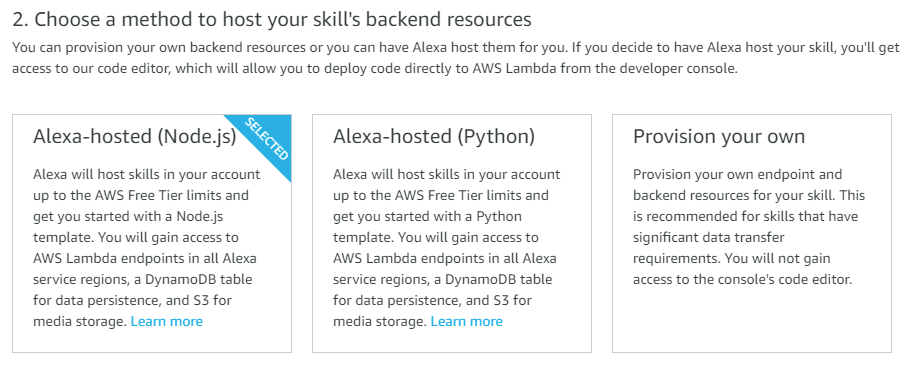
At the top of the same page click on the button Create Skill. It will move to a next screen where you select the template. Depending on the language you decide there are previously defined templates which will bring additional code. In this case we are starting from scratch, however is advisable to experiment the other examples later on this will provide best practices for using features like data persistence, custom intents, session persistence, Alexa conversations, and more.
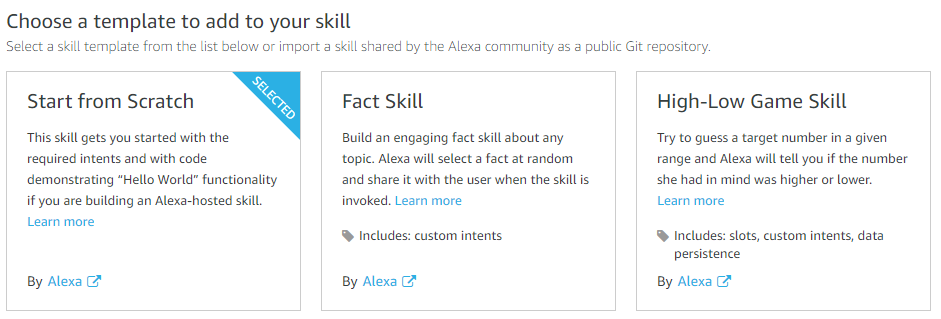
Click on Continue with template, the console will start creating the basic skill automatically.
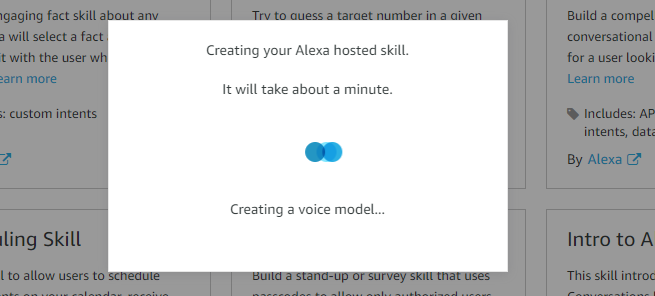
The developer console will go directly to your new skill so you can work on it.
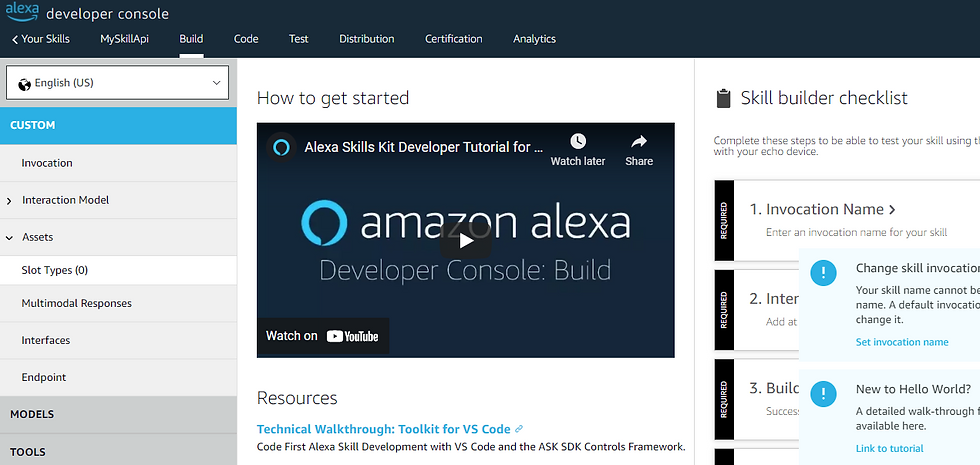
First we need to update the invocation name, this will be how your skill will be called, or started. For instance, if you invocation name is "call api" to start your skill you will have to say to Alexa "open call api". Use small caps when adding this information.
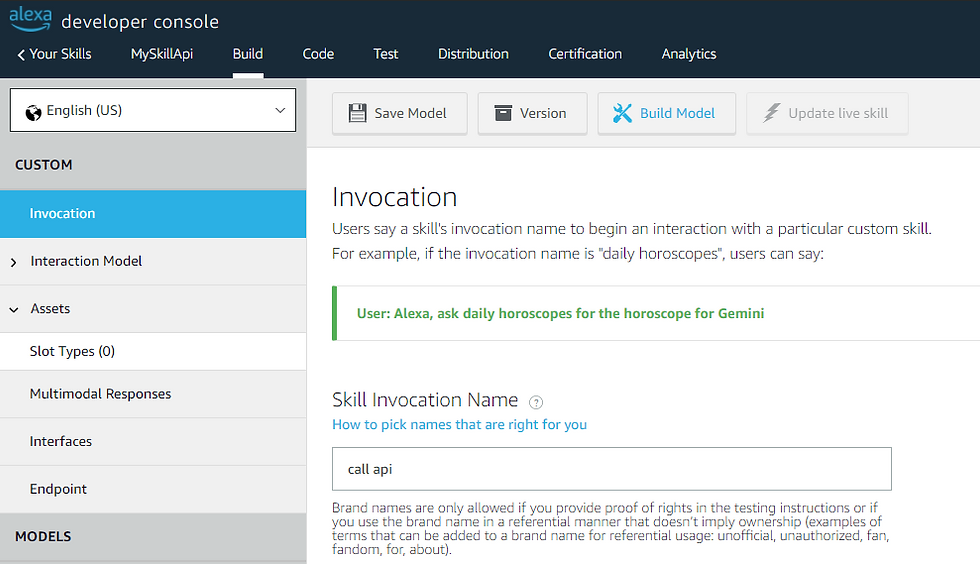
Save the model, using the button in the Invocation page.
In the Invocation page, the template will automatically create a basic intent. The intent represents an action that is associated with a spoken request from the user. Intents can have arguments called slots which we are not covering in this article.
We will be working with the basic intent called HelloWorldIntent. This can be modified at any time. Notice that there Utterances, once you start your skill you will want to provide voice commands to continue the interaction, hence you can have multiple utterances to call the same intent. For testing purposes, we are adding one more utterances as "call my api", as the image below demonstrates.
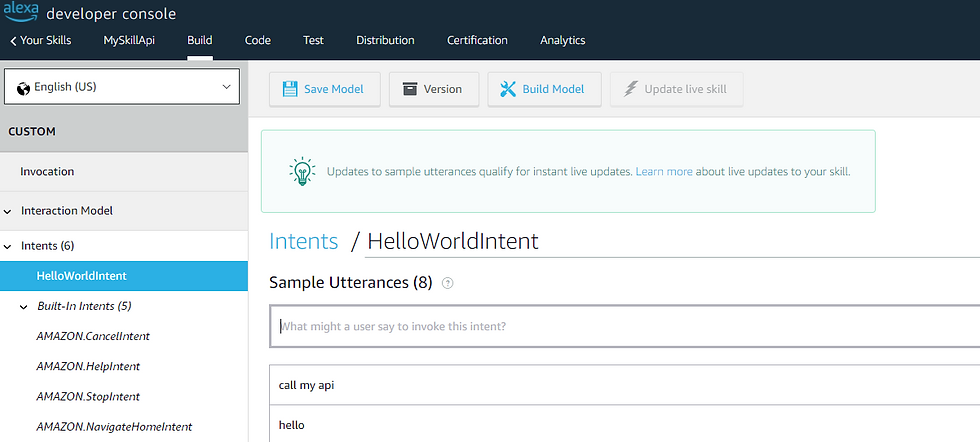
Save the model again.
Build the model, this will take a few seconds to complete a message will appear in the bottom right.
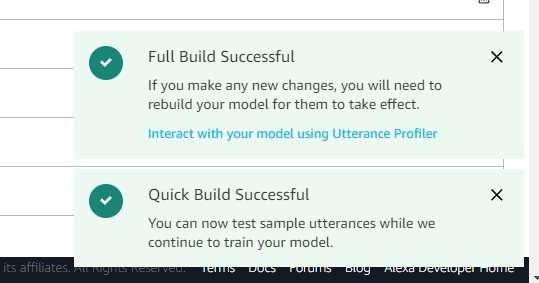
We can test this right away. Click on Test at the top. It will be disabled by default. Change the Off value to Development.
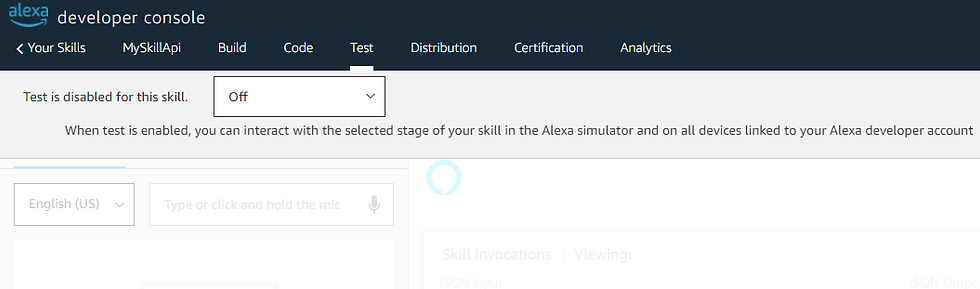
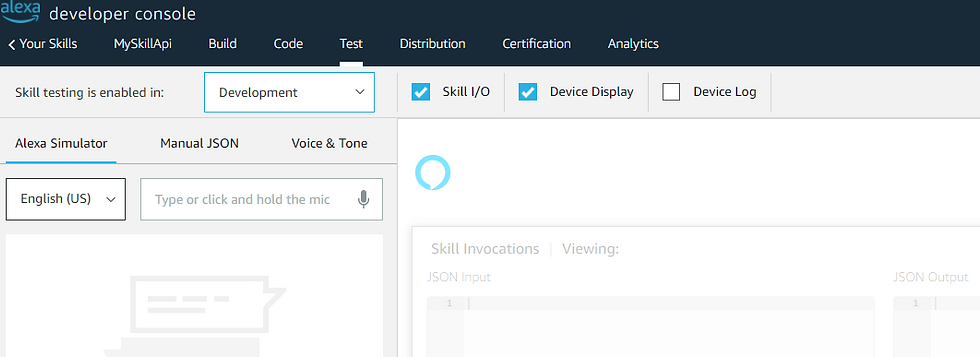
You are ready to interact with this new skill. You might want to allow the browser to access your microfone if you do not want to type the commands all the time. To use the microphone, click and hold the icon button and speak.
To trigger your new skill use the command - "open call api". Notice on the image below that when a successful interpretation of you input (voice or text) will generate a skill invocation call which will have a response if successful. Your skill is now responding.
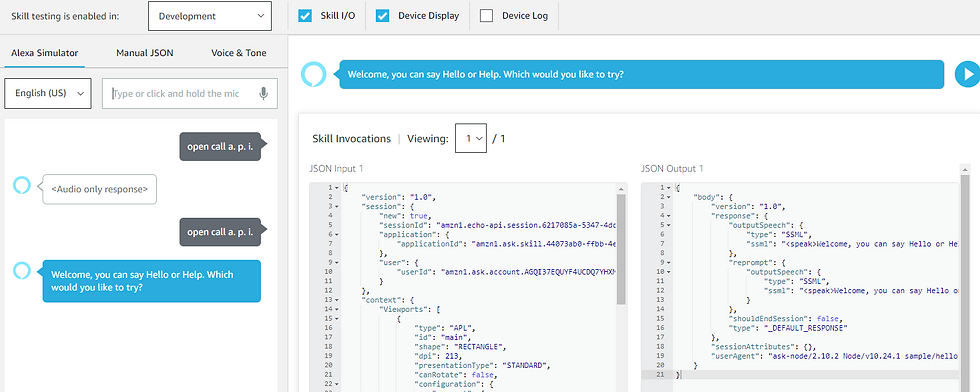
Now we will want to add a feature to it, to make it useful and fun. So we need to get to the coding part. Click on the Code tab of your developer console. This will bring your code editor. The index.js is the main source that will hold all the associations between your intent and the logic that you wish to apply to your skill.
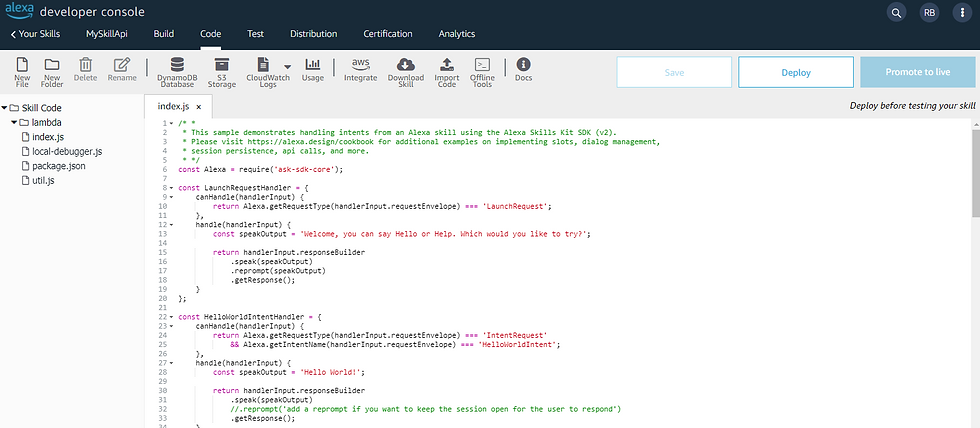
The launch request represents the first interaction from the user when your skill is called. You can use to inform back to the user what is this skill can do. Below we edited the speakOutput constant which will be built into a response from Alexa when returning the handlerInput.
Do this change, save and deploy your change. This will make your simple change available for testing.

Below you can see that the interaction is changed as we change the code. you can also notice that the HelloWorld intent.
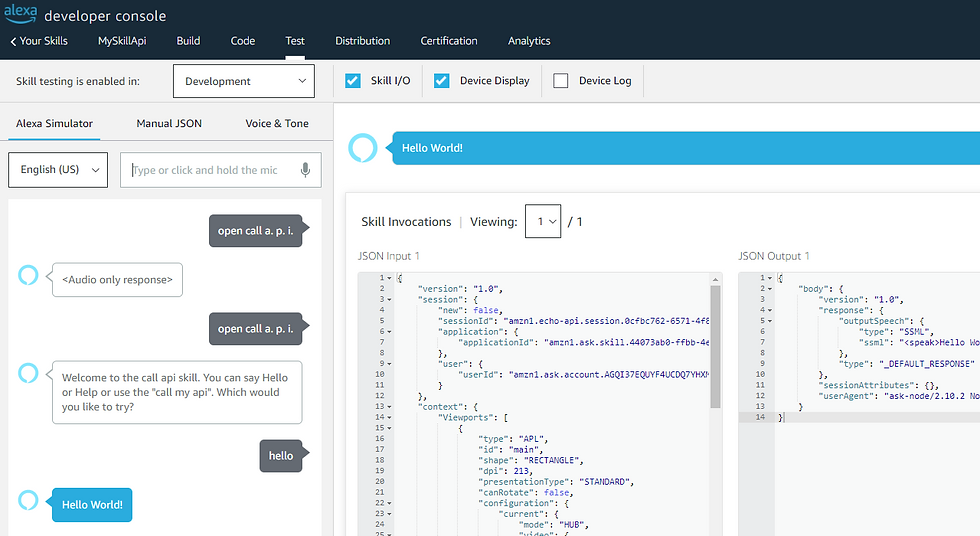
Now we want to step it up a little bit and perform a call to an external service and use it to return the information to the user using Alexa voice.
We will need to a http call to the service hence we need to import the https component which is inside the SDK we are already using
var https = require('https');
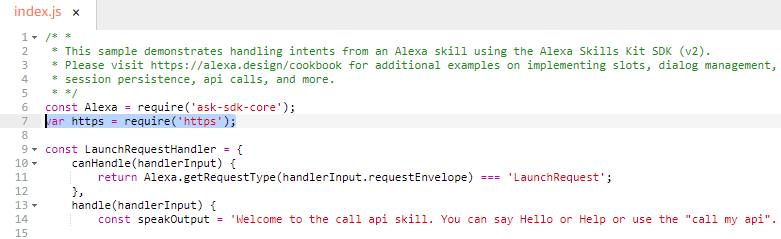
Add the following function to be able to call the external resource. For now it can be located right below your handler and refactored later.
function httpsGet() {
return new Promise(((resolve, reject) => {
var options = {
host: 'api.icndb.com',
port: 443,
path: '/jokes/random',
method: 'GET',
};
const request = https.request(options, (response) => {
response.setEncoding('utf8');
let returnData = '';
response.on('data', (chunk) => {
returnData += chunk;
});
response.on('end', () => {
resolve(JSON.parse(returnData));
});
response.on('error', (error) => {
reject(error);
});
});
request.end();
}));
}
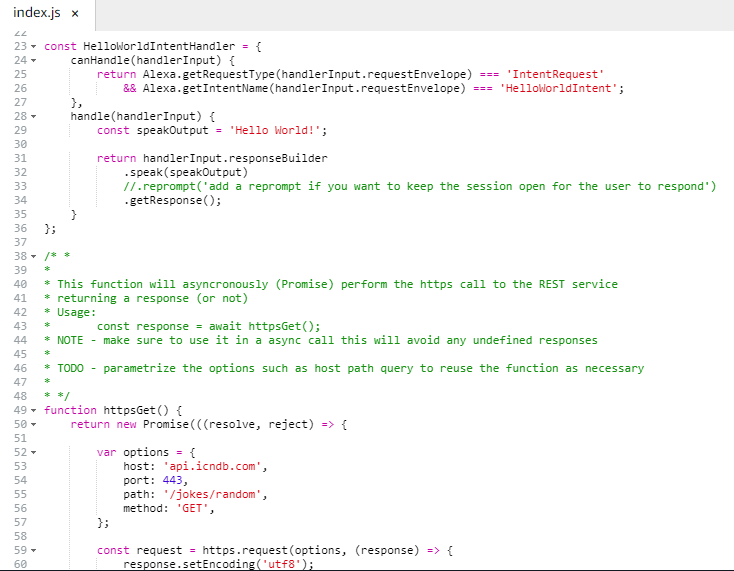
Change your code in the intent handler in order to use the new function.
const HelloWorldIntentHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'IntentRequest'
&& Alexa.getIntentName(handlerInput.requestEnvelope) === 'HelloWorldIntent';
},
async handle(handlerInput) {
//calls the function to fecth the response
//put the response in the variable
const response = await httpsGet();
//logs the response in cloud watch logs
console.log(response);
//return to Alexa for speaking out what you wish
return handlerInput.responseBuilder
.speak("Okay. Here is what I got back from my request. " + response.value.joke)
.reprompt("What would you like?")
.getResponse();
}
};
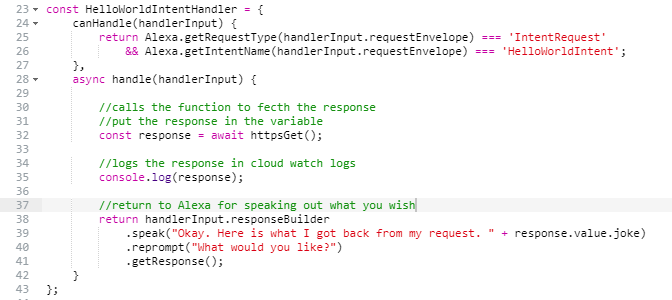
Save, deploy and re-test your skill. The below example we called an API that will return a random joke. This is then picked from the JSON response and used in the response.
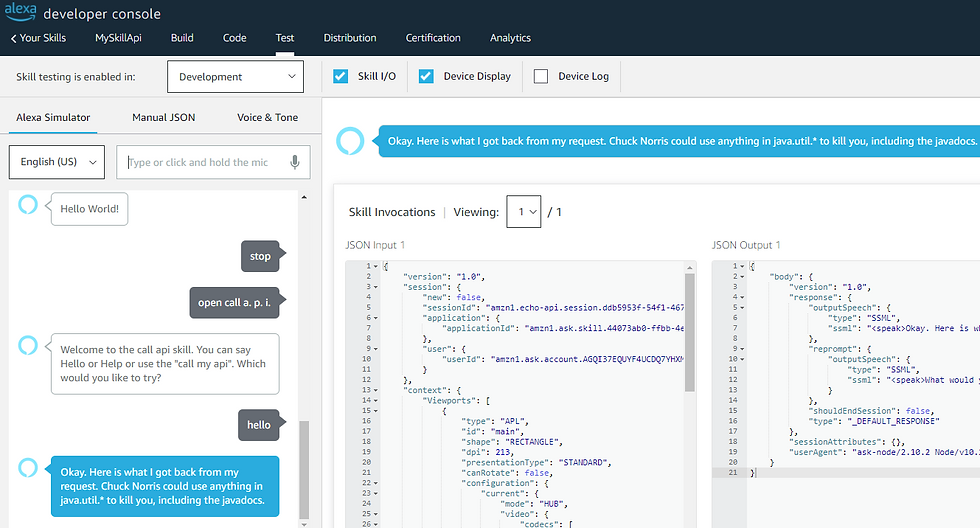
To improve your visibility during development, it is really important to console.log your functions, the logs are sent to cloud watch and are easily accessible using the button on the top of the code console
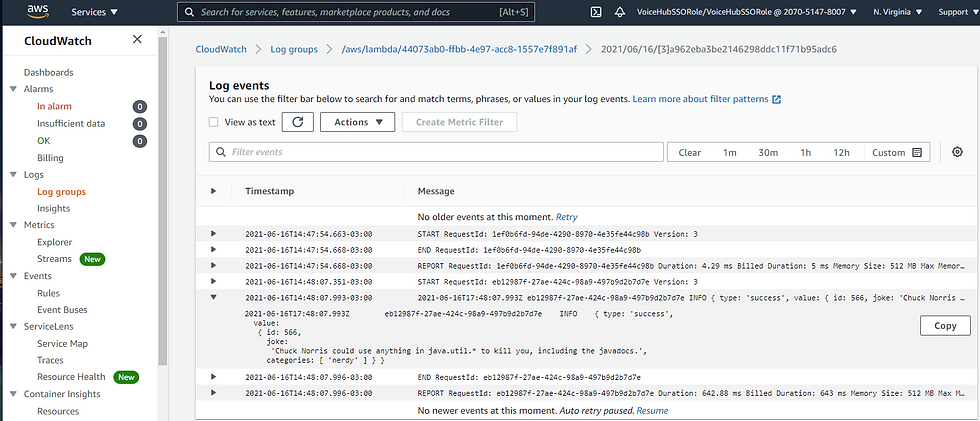
Comments